PV-WAVE Operators
The following types of operators are described in this section:

Assignment, array, numeric, and string operators

Boolean operators

Relational operators
Assignment, Array, and Numeric Operators
Summary of Operators lists the commonly available operators.
Parentheses ( )
Used in grouping of expressions and to enclose subscript and function parameter lists. Parentheses can be used to override order of operator evaluation as described above. Examples:
; Parentheses enclose subscript lists, if A is a variable.
A(X, Y)
; Parentheses enclose function argument lists.
SIN(ANG * PI / 180.)
; Parentheses specify order of operator evaluation.
X = (A + 5) / B
Assignment Operator =
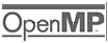
enabled.
The value of the expression on the right side of the equal sign is stored in the variable, subscript element, or range on the left side. For more information, see
"Assignment Statement".
For example:
; Assigns the value of 32 to variable A.
A = 32
Addition Operator +
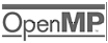
enabled.
Besides arithmetic addition, the addition operator concatenates the strings. For example:
; Assigns the value of 9 to B.
B = 3 + 6
; Assigns the string value of “John Doe” to B.
B = 'John' + ' ' + 'Doe'
See Also
Subtraction Operator –
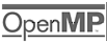
enabled.
Besides subtraction, the minus sign is used as the unary negation operator. For example:
; Assigns the value of 4 to C.
C = 9 - 5
; Changes the sign of C.
C = - C
See Also
Multiplication Operator *
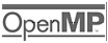
enabled.
Multiplies two operands. For example:
; Assigns the value of 20 to A.
A = 5 * 4
See Also
Division Operator /
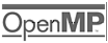
enabled.
Divides two operands. For example:
; Assigns the value of 5 to A.
A = 20 / 4
See Also
Exponentiation Operator ^
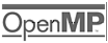
enabled.
A^B is equal to A to the B power. If B is of integer type, repeated multiplication is applied, otherwise the formula AB = e B logA is used. 0^0 is undefined for all operands.
See Also
Matrix Multiplication Operator #
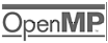
enabled.
note | This operation performs best when the system variable
!CACHE accurately reflects cache sizes on the host machine. |
The rules of linear algebra are followed:

The two operands must conform in that the second dimension of the first operand must equal the first dimension of the second operand.

The first dimension of the result is equal to the first dimension of the first operand and the second dimension of the result is equal to the second dimension of the second operand. For example, multiply a (2,3) matrix by a (3,4) matrix:
a = FINDGEN(2,3) & PM, a
; PV-WAVE prints:
; 0.00000 2.00000 4.00000
; 1.00000 3.00000 5.00000
b = FINDGEN(3,4) & PM, b
; PV-WAVE prints:
; 0.00000 3.00000 6.00000 9.00000
; 1.00000 4.00000 7.00000 10.0000
; 2.00000 5.00000 8.00000 11.0000
c = a # b & PM, c
; PV-WAVE prints:
; 10.0000 28.0000 46.0000 64.0000
; 13.0000 40.0000 67.0000 94.0000
As with other PV-WAVE operators, if the data types of the operands do not match, computations are done with the type yielding the greatest precision. If both operands are of some integer type, computations are done with long integers.
If a parameter is a one-dimensional vector, it is interpreted as either a row or column vector, whichever conforms to the other operand. If both operands are vectors, the result of the operation is the outer product of the two vectors. The inner product of b and c can be computed as REFORM(b,1,N_ELEMENTS(b)) # c or as TOTAL(b*c), although the former is in general more efficient.
For sparse-matrix dense-vector multiplications, see the
SPMVM Function.
See Also
MOD
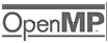
enabled.
Modulo operator. I MOD J is equal to the remainder when I is divided by J, and it is defined when I or J are integer, floating point, double-precision or complex. For example:
; A is set to 4.
A = 9 MOD 5
; Compute angle modulo 2π.
A = (ANGLE + B) MOD (2 * PI)
See Also
Array Concatenation Operators [ ]
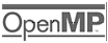
enabled.
Operands enclosed in square brackets and separated by commas are concatenated to form larger arrays. The expression [A, B] is an array formed by concatenating the first dimensions of A and B, which may be scalars or arrays.
Similarly, [A, B, C] concatenates A, B, and C. The second and third dimensions may be concatenated by nesting the bracket levels: [[1, 2], [3, 4]] is a two-by-two array with the first row containing 1 and 2, and the second row containing 3 and 4. Operands must have compatible dimensions: all dimensions must be equal except the dimension that is to be concatenated. For example, [2, INTARR(2, 2)] are incompatible.
For example:
; Defines C as three-point vector.
C = [-1, 1, -1]
; Adds a 12 to the end of C.
C = [C, 12]
; Inserts a 12 at the beginning.
C = [12, C]
; Plots ARR2 appended to the end of ARR1.
PLOT, [ARR1, ARR2]
; Defines a 3-by-3 array.
KER = [[1, 2, 1], [2, 4, 2], [1, 2, 1]]
Boolean Operators
The following boolean operators are available:
Results of relational expressions may be combined into more complex expressions using the Boolean operators AND, OR, NOT, and XOR (exclusive OR). The action of these operators is summarized in
Combining Relational Operators:
NOT is the Boolean inverse and is a unary operator because it only has one operand. NOT true is false and NOT false is true.
AND
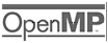
enabled.
AND is the Boolean operator for logical conjunction. It results in true only when both operands are true. This operator works bitwise on all integer data types (i.e. BYTE, INT, INT32, and LONG).
For example:
IF 0 AND 0 THEN PRINT,'true' ELSE PRINT,'false'
IF 0 AND 1 THEN PRINT,'true' ELSE PRINT,'false'
IF 1 AND 0 THEN PRINT,'true' ELSE PRINT,'false'
IF 1 AND 1 THEN PRINT,'true' ELSE PRINT,'false'
See Also
NOT
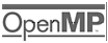
enabled.
NOT is the Boolean complement operator. NOT true is false. NOT complements each bit for all integer operands. For floating point operands, the result is 1.0 if the operand is zero, otherwise, the result is zero. NOT is the Boolean inverse and is a unary operator because it only has one operand. NOT true is false and NOT false is true.
For example:
IF NOT 0 THEN PRINT,'true' ELSE PRINT,'false'
IF NOT 1 THEN PRINT,'true' ELSE PRINT,'false'
See Also
OR
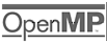
enabled.
OR is the Boolean inclusive operator. For all integer operands a bitwise inclusive “or” is performed. For example, 3 OR 5 equals 7. For floating point operands the OR operator returns the first operand if neither operand is zero, otherwise it returns the second operand.
For example:
IF 0 OR 0 THEN PRINT,'true' ELSE PRINT,'false'
IF 0 OR 1 THEN PRINT,'true' ELSE PRINT,'false'
IF 1 OR 0 THEN PRINT,'true' ELSE PRINT,'false'
IF 1 OR 1 THEN PRINT,'true' ELSE PRINT,'false'
See Also
XOR
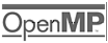
enabled.
The Boolean exclusive “or” function. XOR is only valid for all integer operands. XOR returns a one bit if the corresponding bits in the operands are different; if they are equal, a zero bit is returned.
See Also
For example:
IF 0 XOR 0 THEN PRINT,'true' ELSE PRINT,'false'
IF 0 XOR 1 THEN PRINT,'true' ELSE PRINT,'false'
IF 1 XOR 0 THEN PRINT,'true' ELSE PRINT,'false'
IF 1 XOR 1 THEN PRINT,'true' ELSE PRINT,'false'
Examples
When applied to BYTE, INT, INT32, or LONG operands, the Boolean functions operate on each binary bit.
(1 AND 7) ; Evaluates to 1.
(3 OR 5) ; Evaluates to 7.
(NOT 1) ; Evaluates to –2 (twos-complement arithmetic).
(5 XOR 12) ; Evaluates to 9.
When applied to data types that are not integers, the Boolean operators yield the following results:
; Means the value of the last expression if all expressions are
; true (not zero or not the null string), otherwise false (zero
; or the null string).
OP1 AND OP2
; Means the value of the first expression that is true,
; otherwise false if all expressions are false.
OP1 OR OP2
Some examples of relational and Boolean expressions are:
; True if A is between 25 and 50. If A is an array the result is
; an array of ones and zeroes.
(A LE 50) AND (A GE 25)
; True if A is less than 25 or A is greater than 50. This
; expression is the inverse of the first example.
(A GT 50) OR (A LT 25)
; ANDs the hexadecimal constant FF, (255 in decimal) with the
; array ARR. This masks the lower 8 bits and zeroes the upper
; bits.
ARR AND 'FF'X
Relational Operators
The following relational operators are available:
Relational operators apply a relation to two operands and return a logical value of true or false. The resulting logical value may be used as the predicate in IF, WHILE, or REPEAT statements or may be combined using Boolean operators with other logical values to make more complex expressions. For example:
1 EQ 1
is true, and
1 GT 3
is false.
The rules for evaluating relational expressions with operands of mixed modes are the same as those given above for arithmetic expressions. For example, in the relational expression:
(2 EQ 2.0)
the integer 2 is converted to floating point and compared to the Floating point 2.0. The result of this expression is true which is represented by a floating point 1.0.
The value true is represented by the following:

An odd, non-zero value for BYTE, INT, INT32, and LONG integer data types.

Any non-zero value for single, double-precision and complex floating.

Any non-null string.
Conversely, false is represented as anything that is not true: zero- or even-valued integers; zero-valued floating point quantities; and the null string.
The relational operators return a value of 1 for true and zero for false. The type of the result is determined by the same rules that govern the types of arithmetic expressions. So,
(100. EQ 100.)
is 1.0, while
(100 EQ 100)
is 1, the integer.
Relational operators may be applied to arrays and the result, which is an array of ones and zeroes, may be used as an operand. For example, the expression:
ARR * (ARR LE 100)
is an array equal to ARR except that all points greater than 100 have been zeroed. The expression (ARR LE 100) is an array that contains a 1 where the corresponding element of ARR is less than or equal to 100, and zero otherwise.
Minimum Operator <
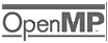
enabled.
The value of A < B is equal to the smaller of A or B. For example:
; Sets A to 3.
A = 5 < 3
; Sets all points in array ARR that are larger than 100 to 100.
ARR = ARR < 100
; Sets X to smallest operand.
X = X0 < X1 < X2
See Also
Maximum Operator >
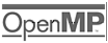
enabled.
A > B is equal to the larger of A or B. For example:
; Avoids taking logs of 0 or negative numbers.
C = ALOG(D > 1E-6)
; Plots only positive points. Negative points are plotted as
; zero.
PLOT, ARR > 0
See Also
EQ
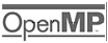
enabled.
EQ returns true if its operands are equal, otherwise it is false. For floating point operands true is 1.000; for integers types, it is 1. For string operands, a zero-length null string represents false.
See Also
GE
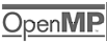
enabled.
GE is the greater than or equal to relational operator. GE returns true if the operand on the left is greater than or equal to the one on the right.
One use of relational operators is to mask arrays:
A = ARRAY * (ARRAY GE 100)
sets A equal to ARRAY whenever the corresponding element of ARRAY is greater than or equal to 100; if the element is less than 100, the corresponding element of A is set to 0.
Strings are compared using the ASCII collating sequence: “ ” is less than “0”, is less than “9”, is less than “A”, is less than “Z”, is less than “a”, which is less than “z”.
See Also
GT
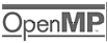
enabled.
Greater than relational operator.
See Also
LE
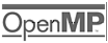
enabled.
Less than or equal to relational operator.
See Also
LT
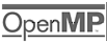
enabled.
Less than relational operator.
See Also
NE
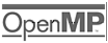
enabled.
NE is the not equal to relational operator. It is true whenever the operands are not of equal value.
See Also
Version 2017.1
Copyright © 2019, Rogue Wave Software, Inc. All Rights Reserved.